머릿속이 너무 복잡해서 공부가 잘 안되네요 오늘은 ㅠㅠ 물론 핑계이지만.. 지금 딴생각이 너무 많네요.
일단 문제 입니다 ^^ 이미지 파일이에용
5.20은 코드가 너무 지저분한 관계로.. 정리가 된 후에 게시하도록 하겠습니다 ㅠ
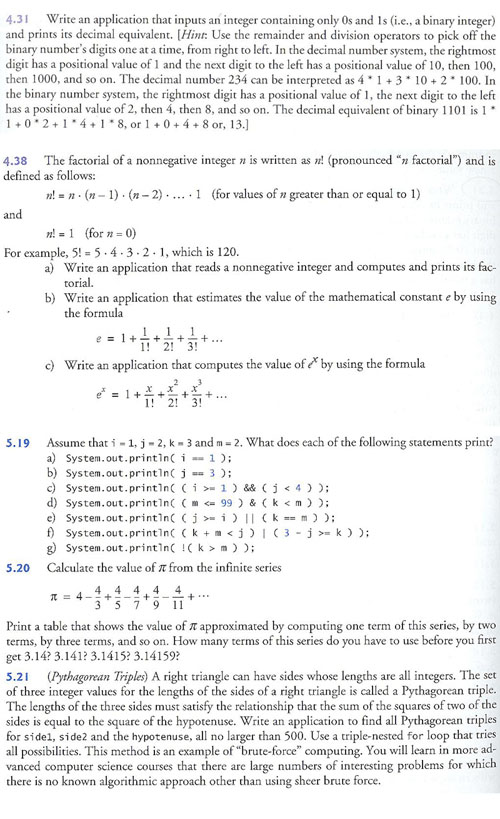
import java.util.Scanner;
public class BinaryToDecimal {
Scanner input = new Scanner(System.in);
private String inputNumber;
private int outputNumber;
// 값을 입력합니다.
private void inputValue() {
System.out.println("변환하실 이진수 값을 입력하세요");
inputNumber = input.next();
}
// 입력된 값을 분석합니다.
private void analyValue(String inputNumber) {
int[] temp = new int[inputNumber.length()];
for (int i = temp.length - 1, j = 0; i > -1; i--, j++) {
Character ch = new Character(inputNumber.charAt(i));
temp[i] = new Integer(ch.toString()) * theSquare(j);
outputNumber += temp[i];
}
}
// 2의 제곱수들을 반환해줍니다.
private int theSquare(int times) {
int base = 1;
if (times !=
0)
for (int i = 1; i <= times; i++)
base = base * 2;
return base;
}
// 실행 시킵니다.
private void run() {
inputValue();
analyValue(inputNumber);
System.out.println(outputNumber);
}
// 메인 메소드
public static void main(String[] args) {
BinaryToDecimal test = new BinaryToDecimal();
test.run();
}
}
public class Factorial {
public int factorial(int num) {
int value = 1;
while (num >
0) {
value = value * num;
num--;
}
return value;
}
public static void main(String[] args) {
Factorial test = new Factorial();
System.out.println(test.factorial(5));
}
}
import java.util.Scanner;
public class AndOrOperator {
private static int i = 1;
private static int j = 2;
private static int k = 3;
private static int m = 2;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Please Insert Alphabet : A to G");
String input = sc.next();
input.toLowerCase();
switch
(input.charAt(0)) {
case 'a':
System.out.println("System.out.println(i
== 1)");
System.out.println(i == 1);
break;
case 'b':
System.out.println("System.out.println(j
== 3)");
System.out.println(j == 3);
break;
case 'c':
System.out.println("System.out.println((i
>= 1) && (j < 4))");
System.out.println((i >= 1) && (j < 4));
break;
case 'd':
System.out.println("System.out.println((m
<= 99) & (k < m))");
System.out.println((m <= 99) & (k < m));
break;
case 'e':
System.out.println("System.out.println((j
>= i) || (k == m))");
System.out.println((j >= i) || (k == m));
break;
case 'f':
System.out
.println("System.out.println((k + m < j) |
(3 - j >= k))");
System.out.println((k + m < j) | (3 - j >= k));
break;
case 'g':
System.out.println("System.out.println(!(k
> m))");
System.out.println(!(k > m));
break;
default:
System.out.println("Wrong
Alphabet!!!");
}
}
}
public class Pythagorean {
public static void main(String[] args) {
int count = 0; // 피타고라스의 정리가 성립되는 경우의 수
// 직각삼각형에서 hypotenuse는 빗변, side1은 다른 한변, side2는 또다는 한변을 의미한다.
for (int side1 = 1; side1 <= 500; side1++)
for (int side2 = 1; side2 <= 500; side2++)
for (int hypotenuse = 1; hypotenuse <= 500;
hypotenuse++)
if ((hypotenuse * hypotenuse) == (side1 * side1)
+
(side2 * side2)) {
System.out.println(side1 * side1 + " + " + side2
* side2 + " = " + hypotenuse *
hypotenuse);
count++;
}
System.out.println(count);
}
}